Java Collection Framework
The Java collections framework provides a set of interfaces and classes to implement various data structures and algorithms.
For example, the LinkedList class of the collections framework provides the implementation of the doubly-linked list data structure.
Interfaces of Collections Framework
The Java collections framework provides various interfaces. These interfaces include several methods to perform different operations on collections.
Collections Framework Vs. Collection Interface
People often get confused between the collections framework and Collection Interface.
The Collection interface is the root interface of the collections framework. The framework includes other interfaces as well: Map and Iterator. These interfaces may also have subinterfaces.
Java Collection Interface
The Collection interface is the root interface of the collections framework hierarchy.
Java does not provide direct implementations of the Collection interface but provides implementations of its subinterfaces like List, Set, and Queue. To learn more, visit: Java Collection Interface
The Collection interface includes sub-interfaces that are implemented by Java classes. Here are the sub interfaces of the Collection Interface:
List Interface
The List interface is an ordered collection that allows us to add and remove elements like an array. To learn more, visit Java List Interface
Set Interface
The Set interface allows us to store elements in different sets similar to the set in mathematics. It cannot have duplicate elements. To learn more, visit Java Set Interface
Queue Interface
The Queue interface is used when we want to store and access elements in a First In, First Out manner. To learn more, visit Java Queue Interface
Java Map Interface
In Java, the Map interface allows elements to be stored in key/value pairs. Keys are unique names that can be used to access a particular element in a map. And, each key has a single value associated with it. To learn more, visit Java Map Interface
Java Iterator Interface
In Java, the Iterator interface provides methods that can be used to access elements of collections. To learn more, visit Java Iterator Interface
Collections API Algorithms
The Java Collections Framework provides a suite of commonly used algorithms such as sorting and searching, encapsulated within the Collections class. While most of these algorithms primarily operate on List objects, some can be applied to various types of collections.
1) Sorting
The sorting algorithm reorders a List so that its elements are in ascending order based on a specified ordering relationship. There are two variations of this operation:
a) Simple Sort: This version takes a List and sorts its elements according to their natural ordering.
b) Comparator Sort: This version takes both a List and a Comparator, sorting the elements based on the provided Comparator.
2) Shuffling
The shuffle algorithm randomizes the order of elements in a List, effectively destroying any existing order. This process uses a source of randomness to ensure that all possible permutations of the list occur with equal probability, given a fair source of randomness. This algorithm is particularly useful for implementing games of chance.
3) Searching
The binary Search algorithm is designed to search for a specified element in a sorted list. There are two forms of this algorithm:
a) Natural Ordering Search: This form takes a List and a search key, assuming the list is sorted in ascending order according to the natural ordering of its elements.
b) Comparator Search: This form takes a List, a search key, and a Comparator, assuming the list is sorted in ascending order according to the specified Comparator.
Before using binary Search, you can use the sort algorithm to ensure the list is appropriately sorted.
4) Composition
The frequency and disjoint algorithms analyze the composition of one or more collections:
a) Frequency: This algorithm counts the number of times a specified element appears in a given collection.
b) Disjoint: This algorithm checks whether two collections have no elements in common, determining if they are disjoint.
5) Min and Max Values
The min and max algorithms return the minimum and maximum elements in a specified collection, respectively. Both operations are available in two forms:
a) Simple Form: This version takes a collection and returns the minimum (or maximum) element based on the natural ordering of its elements.
b) Comparator Form: This version takes a collection and a Comparator, returning the minimum (or maximum) element according to the specified Comparator.
By leveraging these algorithms, developers can efficiently manipulate and analyze collections in Java, making the Java Collections Framework a powerful tool for handling groups of objects.
Benefits of Java Collections
Java Collections offer several advantages that significantly enhance the efficiency and effectiveness of programming in Java:
a) Reduced Code Complexity: Java Collections streamline code by reducing the number of lines needed to perform common tasks, promoting code reusability and simplifying maintenance.
b) Simplified API Design: Designing APIs becomes more straightforward with Java Collections, as they provide a unified framework for managing groups of objects.
c) Ease of Learning: Utilising predefined APIs within Java Collections accelerates the learning curve for new APIs, making it quicker and easier to understand and implement new functionalities.
d) Increased Productivity: Java Collections minimise the effort required by programmers, thereby increasing development speed and reducing the overall time needed for coding tasks. This efficiency boost leads to faster project completion and enhanced productivity.
Conclusion
The Collections in Java is the backbone of the Java Programming Language. Its interfaces and casses allow developers to effortlessly run programs across networks, systems, software and devices.
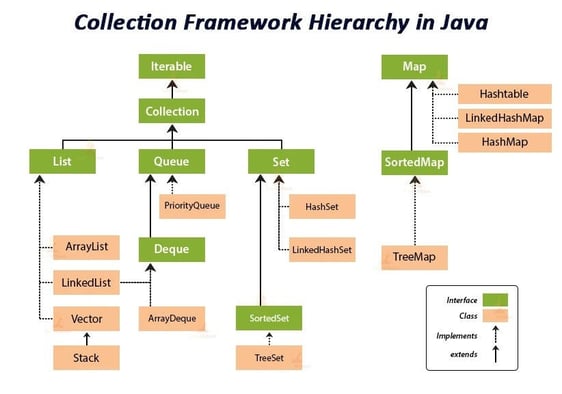
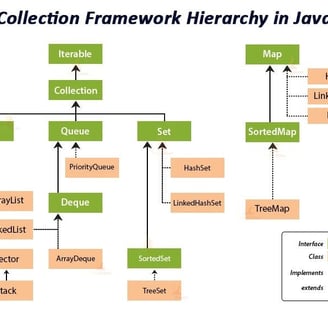
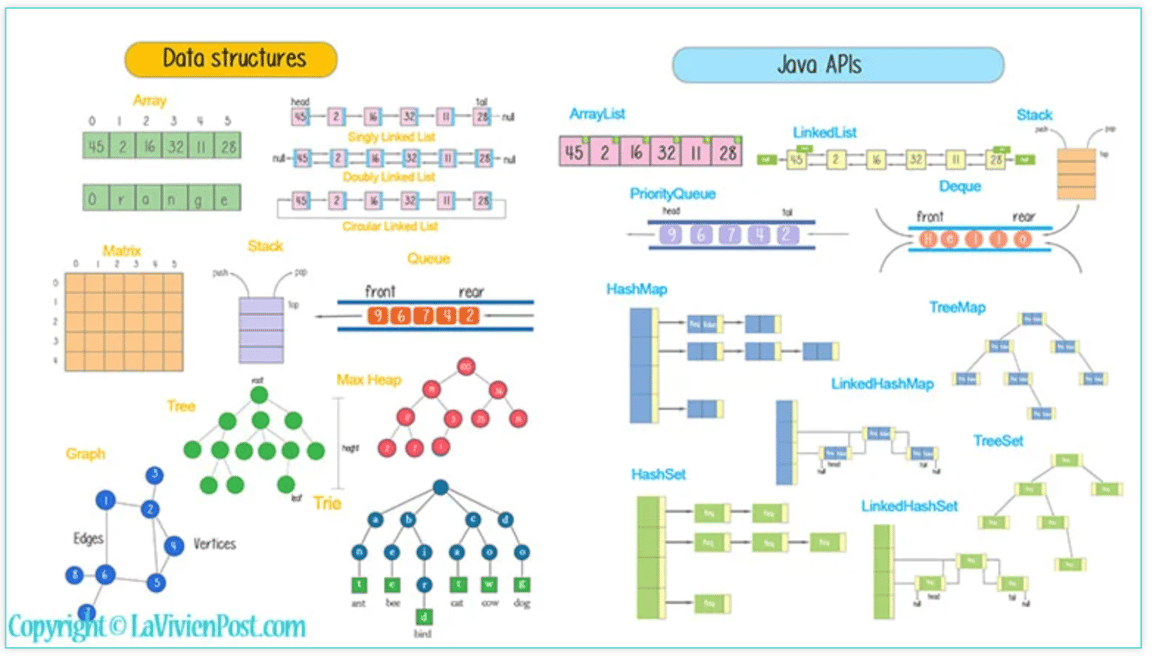
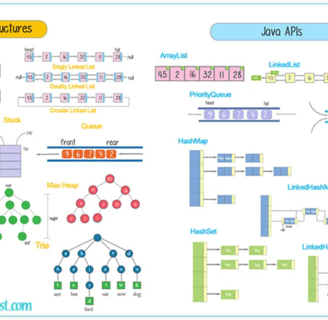
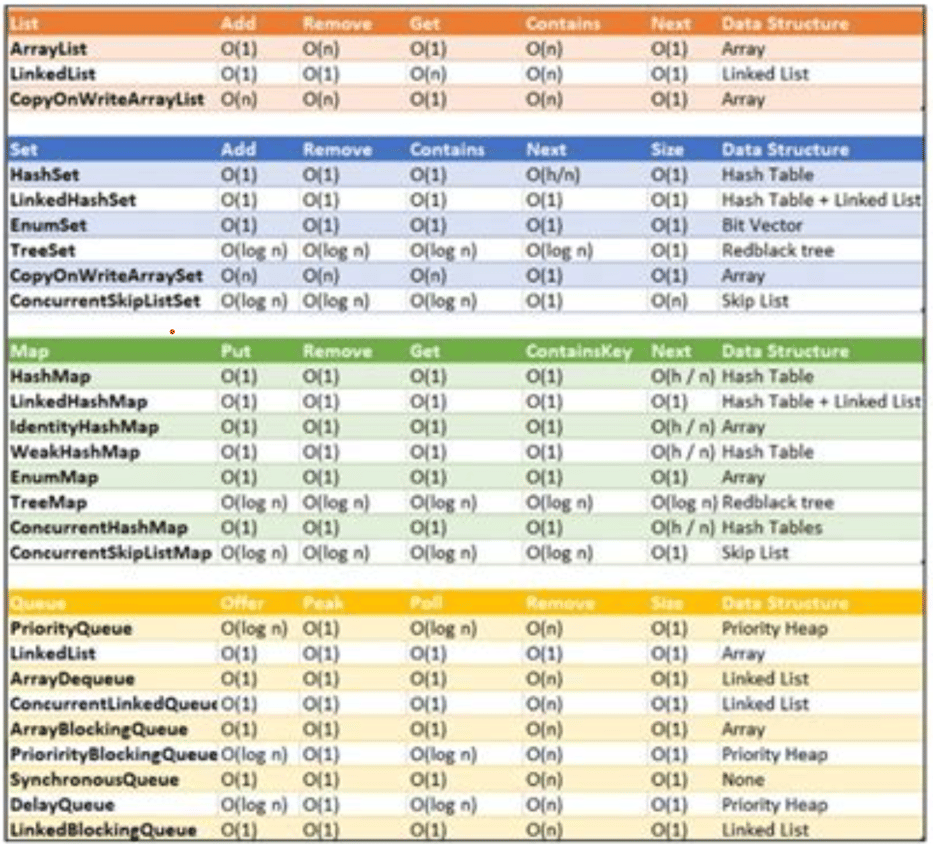
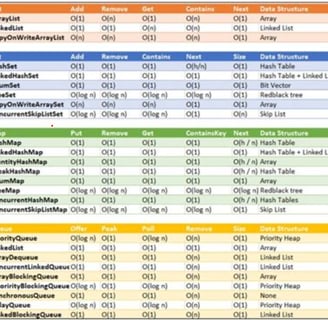
Inspire
Let's Build the Future
Create
youngcrittersacademy@gmail.com
+1 408-768-1983
© 2024. All rights reserved.